DataForm WebPart
1. Open the visual studio.
2. Open the wspbuilder blank webpart solution.
3. Create a class file mentioned in the below image.
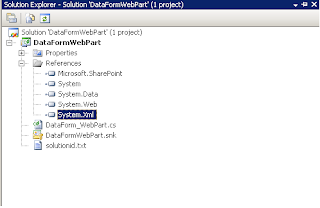
4. Add the reference of micorsoft.Sharepoint, System.xml and System.web as shown in the above image.
5. Create a class file with name "YourClassFileName" but in this case DataForm_webpart.cs
6. Add the Below mentioned code into your file.
---------------------------------------------------------------------------------
using System;
using System.Web;
using System.Xml;
using System.Text;
using System.Web.UI;
using Microsoft.SharePoint;
using System.Web.UI.WebControls;
using Microsoft.SharePoint.WebPartPages;
namespace DataFormWebPart1
{
public class DataForm_WebPart : DataFormWebPart
{
protected override void OnInit(EventArgs e)
{
base.OnInit(e);
Fetch_Data();
}
private void Fetch_Data()
{
StringBuilder strSpCentre = new StringBuilder();
SPSite MySite = SPContext.Current.Site;
SPList list = MySite.RootWeb.GetList("/Lists/yourlistname");
XmlDocument doc = new XmlDocument();
XmlNode queryResponse = doc.AppendChild(doc.CreateElement("dsQueryResponse"));
XmlNode root = queryResponse.AppendChild(doc.CreateElement("Rows"));
XmlElement rowNode;
XmlAttribute att;
if (list != null)
{
foreach (SPListItem item in list.Items)
{
rowNode = doc.CreateElement("Category");
att = doc.CreateAttribute("Title");
att.Value = (string)item["Title"];
rowNode.Attributes.Append(att);
root.AppendChild(rowNode);
att = doc.CreateAttribute("URL");
att.Value = (string)item["Site Url"];
rowNode.Attributes.Append(att);
root.AppendChild(rowNode);
}
}
XmlDataSource source = new XmlDataSource();
source.CacheExpirationPolicy = DataSourceCacheExpiry.Absolute;
source.CacheDuration = 1;
source.Data = doc.InnerXml;
source.ID = "MyTestWebPArts";
this.XslLink = "/Style%20Library/XSL%20Style%20Sheets/DataForm_WebPart_Test.xsl";
this.DataSource = source;
}
public override void DataBind()
{
base.DataBind();
}
}
}
-------------------------------------------------------------------------------------
7. Build the solution. Create wsp file and deploy this wsp file into you webapplication by using central admin.
8. Now open SharePoint 2007 designer here we will create .xsl file which is reffering in the webpart code.
9. Open SharePoint Designer and Create one YourFileName.xsl which is reffering in the above webpart code. Please see below.
10. In my example i have created with DataForm_Webpart_Test.xsl this is i am reffering in my webpart code.
11.Insert the below mentioned code into your xsl file.
------------------------------------------------------------------------
<?xml version="1.0" encoding="utf-8" ?>
<xsl:stylesheet
version="1.0"
exclude-result-prefixes="x d ddwrt xsl msxsl"
xmlns:x="http://www.w3.org/2001/XMLSchema"
xmlns:d="http://schemas.microsoft.com/sharepoint/dsp"
xmlns:ddwrt="http://schemas.microsoft.com/WebParts/v2/DataView/runtime"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform"
xmlns:msxsl="urn:schemas-microsoft-com:xslt">
<xsl:output method="html" indent="no"/>
<xsl:decimal-format NaN=""/>
<xsl:template match="/" xmlns:x="http://www.w3.org/2001/XMLSchema" xmlns:d="http://schemas.microsoft.com/sharepoint/dsp" xmlns:asp="http://schemas.microsoft.com/ASPNET/20" xmlns:__designer="http://schemas.microsoft.com/WebParts/v2/DataView/designer" xmlns:SharePoint="Microsoft.SharePoint.WebControls">
<xsl:call-template name="dvt_1"/>
</xsl:template>
<xsl:template name="dvt_1">
<xsl:variable name="Rows" select="/dsQueryResponse/Rows/Category"></xsl:variable>
<xsl:for-each select="$Rows">
<a href="{@URL}">
<xsl:value-of disable-output-escaping="yes" select="@Title">
</xsl:value-of>
</a>
</xsl:for-each>
</xsl:template>
</xsl:stylesheet>
------------------------------------------------------------------------
12. Save this xsl file and CheckIn and Publish.
13. Pull your webpart from the webpart gallery and put on the page.
14. Now make the changes in the .xsl file according to your requirement.
15 You can do the formating in the .xsl file without touching your webpart.cs file. just open your .xsl file, checkout the file before making any changes. checkin the file and publish with major version. and refresh your webpart page. you will see the changes accrodingly.
16. Please Learn the xslt Syntax from w3schools.com. It will help you to understand the syntax of the xslt.
17. this is a very help full webpart. you can make a very rich UI. according to your requirement with out impacting to the .cs file.
18. In my case my .xsl file is placed in the style library of the site. you can place this file anywhere and just refer the path accordingly.
19. In this example i am reading a list and just displaying the title containing the list item as a link.
1. Open the visual studio.
2. Open the wspbuilder blank webpart solution.
3. Create a class file mentioned in the below image.
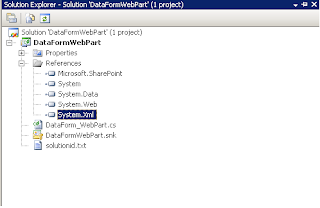
4. Add the reference of micorsoft.Sharepoint, System.xml and System.web as shown in the above image.
5. Create a class file with name "YourClassFileName" but in this case DataForm_webpart.cs
6. Add the Below mentioned code into your file.
---------------------------------------------------------------------------------
using System;
using System.Web;
using System.Xml;
using System.Text;
using System.Web.UI;
using Microsoft.SharePoint;
using System.Web.UI.WebControls;
using Microsoft.SharePoint.WebPartPages;
namespace DataFormWebPart1
{
public class DataForm_WebPart : DataFormWebPart
{
protected override void OnInit(EventArgs e)
{
base.OnInit(e);
Fetch_Data();
}
private void Fetch_Data()
{
StringBuilder strSpCentre = new StringBuilder();
SPSite MySite = SPContext.Current.Site;
SPList list = MySite.RootWeb.GetList("/Lists/yourlistname");
XmlDocument doc = new XmlDocument();
XmlNode queryResponse = doc.AppendChild(doc.CreateElement("dsQueryResponse"));
XmlNode root = queryResponse.AppendChild(doc.CreateElement("Rows"));
XmlElement rowNode;
XmlAttribute att;
if (list != null)
{
foreach (SPListItem item in list.Items)
{
rowNode = doc.CreateElement("Category");
att = doc.CreateAttribute("Title");
att.Value = (string)item["Title"];
rowNode.Attributes.Append(att);
root.AppendChild(rowNode);
att = doc.CreateAttribute("URL");
att.Value = (string)item["Site Url"];
rowNode.Attributes.Append(att);
root.AppendChild(rowNode);
}
}
XmlDataSource source = new XmlDataSource();
source.CacheExpirationPolicy = DataSourceCacheExpiry.Absolute;
source.CacheDuration = 1;
source.Data = doc.InnerXml;
source.ID = "MyTestWebPArts";
this.XslLink = "/Style%20Library/XSL%20Style%20Sheets/DataForm_WebPart_Test.xsl";
this.DataSource = source;
}
public override void DataBind()
{
base.DataBind();
}
}
}
-------------------------------------------------------------------------------------
7. Build the solution. Create wsp file and deploy this wsp file into you webapplication by using central admin.
8. Now open SharePoint 2007 designer here we will create .xsl file which is reffering in the webpart code.
9. Open SharePoint Designer and Create one YourFileName.xsl which is reffering in the above webpart code. Please see below.
10. In my example i have created with DataForm_Webpart_Test.xsl this is i am reffering in my webpart code.
11.Insert the below mentioned code into your xsl file.
------------------------------------------------------------------------
<?xml version="1.0" encoding="utf-8" ?>
<xsl:stylesheet
version="1.0"
exclude-result-prefixes="x d ddwrt xsl msxsl"
xmlns:x="http://www.w3.org/2001/XMLSchema"
xmlns:d="http://schemas.microsoft.com/sharepoint/dsp"
xmlns:ddwrt="http://schemas.microsoft.com/WebParts/v2/DataView/runtime"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform"
xmlns:msxsl="urn:schemas-microsoft-com:xslt">
<xsl:output method="html" indent="no"/>
<xsl:decimal-format NaN=""/>
<xsl:template match="/" xmlns:x="http://www.w3.org/2001/XMLSchema" xmlns:d="http://schemas.microsoft.com/sharepoint/dsp" xmlns:asp="http://schemas.microsoft.com/ASPNET/20" xmlns:__designer="http://schemas.microsoft.com/WebParts/v2/DataView/designer" xmlns:SharePoint="Microsoft.SharePoint.WebControls">
<xsl:call-template name="dvt_1"/>
</xsl:template>
<xsl:template name="dvt_1">
<xsl:variable name="Rows" select="/dsQueryResponse/Rows/Category"></xsl:variable>
<xsl:for-each select="$Rows">
<a href="{@URL}">
<xsl:value-of disable-output-escaping="yes" select="@Title">
</xsl:value-of>
</a>
</xsl:for-each>
</xsl:template>
</xsl:stylesheet>
------------------------------------------------------------------------
12. Save this xsl file and CheckIn and Publish.
13. Pull your webpart from the webpart gallery and put on the page.
14. Now make the changes in the .xsl file according to your requirement.
15 You can do the formating in the .xsl file without touching your webpart.cs file. just open your .xsl file, checkout the file before making any changes. checkin the file and publish with major version. and refresh your webpart page. you will see the changes accrodingly.
16. Please Learn the xslt Syntax from w3schools.com. It will help you to understand the syntax of the xslt.
17. this is a very help full webpart. you can make a very rich UI. according to your requirement with out impacting to the .cs file.
18. In my case my .xsl file is placed in the style library of the site. you can place this file anywhere and just refer the path accordingly.
19. In this example i am reading a list and just displaying the title containing the list item as a link.